Obtain a discharge measurement over a cross section#
Now that we have a suitable velocimetry result, we can extract river flows from it over user-provided cross sections. These must be provided in the same vertical reference as the water level was measured in, and they must eityher be in the same coordinate reference system (crs) as the ground control point observations used in the CameraConfig
object, or in a crs that can be readily transformed into the crs of the camera projection. Below we first read the results back in memory
[1]:
import xarray as xr
import pandas as pd
import pyorc
import cartopy.crs as ccrs
import matplotlib.pyplot as plt
from matplotlib.colors import Normalize
First we read the masked results from notebook 03 back into memory. We also load the first frame of our original video in rgb format.
[2]:
ds = xr.open_dataset("ngwerere/ngwerere_masked.nc")
# also open the original video file
video_file = "ngwerere/ngwerere_20191103.mp4"
video = pyorc.Video(video_file, start_frame=0, end_frame=1)
# borrow the camera config from the velocimetry results
video.camera_config = ds.velocimetry.camera_config
# get the frame as rgb
da_rgb = video.get_frames(method="rgb")
Scanning video: 100%|██████████| 2/2 [00:00<00:00, 18.21it/s]
We need a cross section. We have stored two cross sections in comma-separated text files (.csv) along with the examples. Below we load these in memory and we extract the x, y and z values from it. These are all measured in the same vertical reference as the water level. Let’s first investigate if the cross sections are correctly referenced, by plotting them in the camera configuration.
[3]:
cross_section = pd.read_csv("ngwerere/ngwerere_cross_section.csv")
x = cross_section["x"]
y = cross_section["y"]
z = cross_section["z"]
cross_section2 = pd.read_csv("ngwerere/ngwerere_cross_section_2.csv")
x2 = cross_section2["x"]
y2 = cross_section2["y"]
z2 = cross_section2["z"]
# let's have a look at the cross sections, the coordinates of the cross sections are in UTM 35S coordinates,
# so we have to tell the axes that the coordinates need to be transformed from that crs into the crs of the axes.
# we also make a very very small buffer of 0.00005 degrees around the area of interest, so that we can
# clearly see the cross sections.
ax = ds.velocimetry.camera_config.plot(tiles="GoogleTiles", zoom_level=22, tiles_kwargs={"style": "satellite"}, buffer=0.00005)
ax.plot(x, y, "g--", transform=ccrs.UTM(zone=35, southern_hemisphere=True), label="Cross section #1")
ax.plot(x2, y2, "y--", transform=ccrs.UTM(zone=35, southern_hemisphere=True), label="Cross section #2")
ax.legend()
[3]:
<matplotlib.legend.Legend at 0x7fd08c22e5c0>
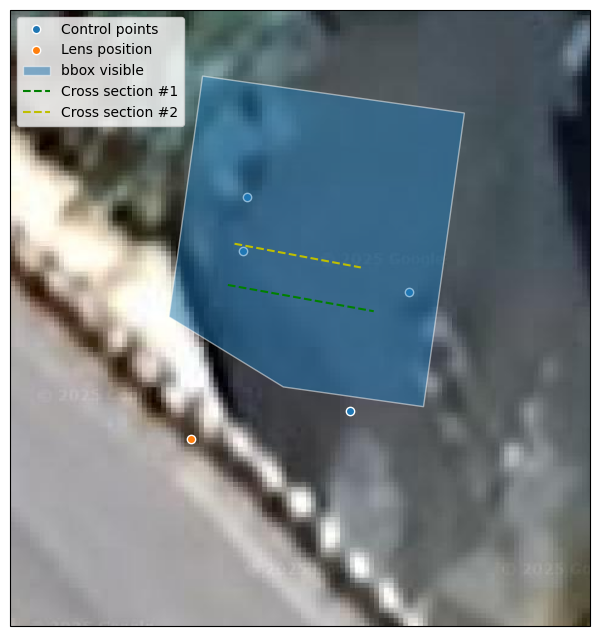
The cross sections are clearly in the area of interest, this means we can sample velocities from them. We use the x, y and z values, to get a transect of velocity values from the velocimetry results. The z-values are only used later, to fill in any missing velocities with a logarithmic profile. The wdw
parameter is used to take the median of the velocity in a larger window. wdw=1
is the default and means that a surrounding window of 3x3 is used to sample from. wdw=0
means that only
the pixel directly underneath the bathymetry point is used. To account for short duration variability in FPS or natural occurring velocities, we apply a little bit of smoothing in time on the results with a rolling mean over 4 time steps as a setting.
[4]:
ds_points = ds.velocimetry.get_transect(x, y, z, crs=32735, rolling=4)
ds_points2 = ds.velocimetry.get_transect(x2, y2, z2, crs=32735, rolling=4)
ds_points
[4]:
<xarray.Dataset> Size: 13kB Dimensions: (points: 39, quantile: 5) Coordinates: (12/13) xp (points) float64 312B 492.0 515.7 ... 1.235e+03 1.252e+03 yp (points) float64 312B 287.1 294.1 301.1 ... 502.5 507.6 512.6 xs (points) float64 312B 6.427e+05 6.427e+05 ... 6.427e+05 ys (points) float64 312B 8.304e+06 8.304e+06 ... 8.304e+06 lon (points) float64 312B 28.33 28.33 28.33 ... 28.33 28.33 28.33 lat (points) float64 312B -15.33 -15.33 -15.33 ... -15.33 -15.33 ... ... y (points) float64 312B 6.166 6.036 5.906 ... 1.489 1.359 1.229 xcoords (points) float64 312B 6.427e+05 6.427e+05 ... 6.427e+05 ycoords (points) float64 312B 8.304e+06 8.304e+06 ... 8.304e+06 scoords (points) float64 312B 0.0 0.13 0.26 0.39 ... 4.68 4.81 4.94 zcoords (points) float64 312B 1.182e+03 1.182e+03 ... 1.183e+03 * quantile (quantile) float64 40B 0.05 0.25 0.5 0.75 0.95 Dimensions without coordinates: points Data variables: v_x (quantile, points) float64 2kB nan nan nan nan ... nan nan nan v_y (quantile, points) float64 2kB nan nan nan nan ... nan nan nan s2n (quantile, points) float64 2kB nan nan nan nan ... nan nan nan corr (quantile, points) float64 2kB nan nan nan nan ... nan nan nan cols (points) float64 312B 19.93 19.89 19.85 ... 18.57 18.53 18.5 rows (points) float64 312B 11.57 12.57 13.57 ... 47.55 48.55 49.54 v_dir (points) float64 312B -4.676 -4.676 -4.674 ... -4.676 -4.676 v_eff_nofill (quantile, points) float64 2kB nan nan nan nan ... nan nan nan Attributes: camera_shape: [1080, 1920] camera_config: {\n "height": 1080,\n "width": 1920,\n "crs": "P... h_a: 0.0
- points: 39
- quantile: 5
- xp(points)float64492.0 515.7 ... 1.235e+03 1.252e+03
- axis :
- X
- long_name :
- column in camera perspective
- units :
- -
array([ 491.96849675, 515.74554673, 539.28089429, 562.59440578, 585.68411844, 608.49303463, 631.10424013, 653.49803912, 675.6724587 , 697.63593419, 719.37812275, 740.9083282 , 762.22172734, 783.30634041, 804.21224281, 824.92321424, 845.43781861, 865.75368875, 885.882615 , 905.85033113, 925.60559226, 945.17026965, 964.55712362, 983.7584016 , 1002.79958346, 1021.67840564, 1040.37693127, 1058.89880116, 1077.2551038 , 1095.45649908, 1113.51966885, 1131.43219625, 1149.14597885, 1166.70405633, 1184.10847661, 1201.3612775 , 1218.46438105, 1235.4197276 , 1252.22926685])
- yp(points)float64287.1 294.1 301.1 ... 507.6 512.6
- axis :
- Y
- long_name :
- row in camera perspective
- units :
- -
array([287.06069404, 294.12937077, 301.12665793, 308.00211169, 314.77882468, 321.63961476, 328.34420774, 334.96503092, 341.51857452, 347.99238053, 354.42323554, 360.79505306, 367.12747981, 373.45527629, 379.66648101, 385.80903619, 391.89373753, 397.93248118, 403.90615475, 409.76799494, 415.63224803, 421.45758939, 427.22387032, 432.95168168, 438.59757363, 444.17284166, 449.7162139 , 455.22455046, 460.68210629, 466.07543954, 471.38065192, 476.62499604, 481.89287494, 487.11479597, 492.29139165, 497.42320476, 502.51084915, 507.55486587, 512.55581921])
- xs(points)float646.427e+05 6.427e+05 ... 6.427e+05
- axis :
- X
- long_name :
- x-coordinate in Cartesian system
- units :
- m
array([642732.215 , 642732.3428526 , 642732.47070519, 642732.59849397, 642732.72624444, 642732.85418309, 642732.98201246, 642733.10981936, 642733.23762293, 642733.36540564, 642733.4932139 , 642733.62102593, 642733.74886636, 642733.87678068, 642734.00462051, 642734.13244717, 642734.26027383, 642734.38811566, 642734.51594538, 642734.64369599, 642734.77152604, 642734.89937774, 642735.02722148, 642735.15508551, 642735.28290656, 642735.41070432, 642735.53853566, 642735.66638825, 642735.79423836, 642735.92206502, 642736.04983048, 642736.17757371, 642736.30542262, 642736.43327154, 642736.56112045, 642736.68896937, 642736.81681828, 642736.9446672 , 642737.07251611])
- ys(points)float648.304e+06 8.304e+06 ... 8.304e+06
- axis :
- Y
- long_name :
- y-coordinate in Cartesian system
- units :
- m
array([8304295.84700001, 8304295.82346886, 8304295.7999377 , 8304295.77606557, 8304295.75199084, 8304295.72893213, 8304295.70527676, 8304295.68149873, 8304295.65770282, 8304295.63379509, 8304295.61002435, 8304295.58627388, 8304295.56267809, 8304295.53948484, 8304295.51588511, 8304295.4922135 , 8304295.4685419 , 8304295.4449524 , 8304295.42129827, 8304295.39721962, 8304295.37356803, 8304295.35003216, 8304295.32645304, 8304295.30298416, 8304295.27928225, 8304295.25545522, 8304295.23180941, 8304295.20827826, 8304295.18473366, 8304295.16106206, 8304295.13706372, 8304295.11294697, 8304295.08939586, 8304295.06584474, 8304295.04229363, 8304295.01874251, 8304294.9951914 , 8304294.97164028, 8304294.94808916])
- lon(points)float6428.33 28.33 28.33 ... 28.33 28.33
- long_name :
- longitude
- units :
- degrees_east
array([28.32963233, 28.32963352, 28.32963471, 28.3296359 , 28.32963709, 28.32963829, 28.32963948, 28.32964067, 28.32964186, 28.32964305, 28.32964424, 28.32964544, 28.32964663, 28.32964782, 28.32964901, 28.3296502 , 28.3296514 , 28.32965259, 28.32965378, 28.32965497, 28.32965616, 28.32965736, 28.32965855, 28.32965974, 28.32966093, 28.32966212, 28.32966332, 28.32966451, 28.3296657 , 28.32966689, 28.32966808, 28.32966927, 28.32967047, 28.32967166, 28.32967285, 28.32967404, 28.32967523, 28.32967643, 28.32967762])
- lat(points)float64-15.33 -15.33 ... -15.33 -15.33
- long_name :
- latitude
- units :
- degrees_north
array([-15.33397933, -15.33397954, -15.33397974, -15.33397995, -15.33398016, -15.33398037, -15.33398057, -15.33398078, -15.33398099, -15.3339812 , -15.3339814 , -15.33398161, -15.33398182, -15.33398202, -15.33398223, -15.33398243, -15.33398264, -15.33398285, -15.33398305, -15.33398326, -15.33398347, -15.33398368, -15.33398388, -15.33398409, -15.33398429, -15.3339845 , -15.33398471, -15.33398492, -15.33398512, -15.33398533, -15.33398554, -15.33398575, -15.33398595, -15.33398616, -15.33398637, -15.33398657, -15.33398678, -15.33398698, -15.33398719])
- x(points)float642.655 2.65 2.646 ... 2.474 2.469
array([2.65525023, 2.65048555, 2.64572086, 2.64060955, 2.63529222, 2.63100746, 2.62611651, 2.62110093, 2.61606717, 2.61091975, 2.60591159, 2.60092403, 2.59609362, 2.59167221, 2.58683782, 2.58193041, 2.57702299, 2.57219901, 2.56730934, 2.56198816, 2.55710103, 2.55233155, 2.54751812, 2.54281671, 2.53787849, 2.53281311, 2.5279319 , 2.52316721, 2.51838886, 2.51348145, 2.50824189, 2.50288194, 2.49809697, 2.493312 , 2.48852702, 2.48374205, 2.47895708, 2.47417211, 2.46938714])
- y(points)float646.166 6.036 5.906 ... 1.359 1.229
array([6.16567471, 6.03576206, 5.9058494 , 5.77595049, 5.64606014, 5.51613078, 5.38622311, 5.25631989, 5.1264174 , 4.99651934, 4.86661585, 4.73671156, 4.60680158, 4.47687679, 4.34696682, 4.21705948, 4.08715214, 3.95724169, 3.82733385, 3.6974428 , 3.56753501, 3.43762255, 3.3077117 , 3.17779674, 3.04789057, 2.91798931, 2.78808107, 2.65816842, 2.52825627, 2.39834893, 2.26845483, 2.13856556, 2.00865365, 1.87874174, 1.74882983, 1.61891792, 1.48900601, 1.35909411, 1.2291822 ])
- xcoords(points)float646.427e+05 6.427e+05 ... 6.427e+05
array([642732.215 , 642732.34285259, 642732.47070518, 642732.59849397, 642732.72624444, 642732.85418308, 642732.98201245, 642733.10981936, 642733.23762293, 642733.36540563, 642733.49321389, 642733.62102592, 642733.74886635, 642733.87678067, 642734.0046205 , 642734.13244716, 642734.26027382, 642734.38811565, 642734.51594537, 642734.64369598, 642734.77152603, 642734.89937773, 642735.02722147, 642735.1550855 , 642735.28290654, 642735.41070431, 642735.53853565, 642735.66638824, 642735.79423835, 642735.92206501, 642736.04983046, 642736.17757369, 642736.30542261, 642736.43327152, 642736.56112044, 642736.68896935, 642736.81681827, 642736.94466718, 642737.0725161 ])
- ycoords(points)float648.304e+06 8.304e+06 ... 8.304e+06
array([8304295.847 , 8304295.82346885, 8304295.7999377 , 8304295.77606556, 8304295.75199083, 8304295.72893212, 8304295.70527675, 8304295.68149872, 8304295.65770281, 8304295.63379508, 8304295.61002434, 8304295.58627387, 8304295.56267808, 8304295.53948482, 8304295.51588509, 8304295.49221349, 8304295.46854188, 8304295.44495238, 8304295.42129826, 8304295.39721961, 8304295.37356801, 8304295.35003214, 8304295.32645302, 8304295.30298415, 8304295.27928223, 8304295.2554552 , 8304295.23180939, 8304295.20827824, 8304295.18473364, 8304295.16106204, 8304295.1370637 , 8304295.11294695, 8304295.08939584, 8304295.06584472, 8304295.0422936 , 8304295.01874249, 8304294.99519137, 8304294.97164026, 8304294.94808914])
- scoords(points)float640.0 0.13 0.26 ... 4.68 4.81 4.94
array([0. , 0.13, 0.26, 0.39, 0.52, 0.65, 0.78, 0.91, 1.04, 1.17, 1.3 , 1.43, 1.56, 1.69, 1.82, 1.95, 2.08, 2.21, 2.34, 2.47, 2.6 , 2.73, 2.86, 2.99, 3.12, 3.25, 3.38, 3.51, 3.64, 3.77, 3.9 , 4.03, 4.16, 4.29, 4.42, 4.55, 4.68, 4.81, 4.94])
- zcoords(points)float641.182e+03 1.182e+03 ... 1.183e+03
array([1182.3 , 1182.11724139, 1182.04766272, 1182.02136012, 1182.00426783, 1182.0288142 , 1182.05395595, 1182.07886069, 1182.1 , 1182.1 , 1182.1 , 1182.1 , 1182.1 , 1182.1 , 1182.07344832, 1182.04145034, 1182.00995529, 1181.97707483, 1181.9434698 , 1181.91002723, 1181.91869847, 1181.94585887, 1181.97331208, 1182. , 1182. , 1182. , 1182.07595305, 1182.19360881, 1182.30485649, 1182.35558135, 1182.40611729, 1182.45654225, 1182.50700892, 1182.5574756 , 1182.60794228, 1182.65840895, 1182.70887563, 1182.75934231, 1182.80980899])
- quantile(quantile)float640.05 0.25 0.5 0.75 0.95
array([0.05, 0.25, 0.5 , 0.75, 0.95])
- v_x(quantile, points)float64nan nan nan nan ... nan nan nan nan
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
array([[ nan, nan, nan, nan, 0.08639699, nan, nan, nan, nan, nan, nan, 0.17031846, 0.21440701, 0.22673029, 0.23322227, 0.25430978, 0.26647386, 0.22043808, 0.16107555, 0.07991675, 0.17314457, 0.16577959, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.31837207, nan, nan, nan, nan, nan, nan, 0.21024651, 0.24013688, 0.27037976, 0.26929555, 0.30550776, 0.33299914, 0.30500927, 0.26551842, 0.20316452, 0.23810597, 0.22829523, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.47957168, nan, nan, nan, nan, nan, nan, 0.23214022, 0.2759091 , 0.31364251, 0.31030323, 0.35949721, 0.41953946, 0.41225203, 0.36440482, 0.29994709, 0.28550313, 0.26278804, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.57224314, nan, nan, nan, nan, nan, nan, 0.26305541, 0.30719195, 0.38409759, 0.40652433, 0.4254107 , 0.47594587, 0.48711336, 0.42244001, 0.35852898, 0.34720895, 0.29926047, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.6585597 , nan, nan, nan, nan, nan, nan, 0.2943483 , 0.37690303, 0.4610435 , 0.49172284, 0.49219947, 0.5194662 , 0.53301662, 0.49542274, 0.44170202, 0.40799705, 0.35017769, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]])
- v_y(quantile, points)float64nan nan nan nan ... nan nan nan nan
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
array([[ nan, nan, nan, nan, -0.04975522, nan, nan, nan, nan, nan, nan, 0.02938081, 0.01370522, -0.04768946, -0.02577469, 0.0382964 , 0.04458772, 0.03534167, 0.00577576, -0.12919334, -0.21847965, -0.27601106, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, -0.01597724, nan, nan, nan, nan, nan, nan, 0.04848941, 0.03897506, -0.00104529, 0.01499823, 0.06546107, 0.0754373 , 0.06726933, 0.02845647, -0.07058136, -0.17613938, -0.214507 , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.01317713, nan, nan, nan, nan, nan, nan, 0.0646425 , 0.05165906, 0.02649235, 0.04581243, 0.09939644, 0.10987008, 0.08893217, 0.0497153 , -0.02014032, -0.14401374, -0.18181562, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.06347002, nan, nan, nan, nan, nan, nan, 0.08752184, 0.06515682, 0.04584315, 0.07429326, 0.13336247, 0.15506902, 0.12171582, 0.07211554, 0.01476788, -0.10475965, -0.1569285 , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.16943065, nan, nan, nan, nan, nan, nan, 0.1353601 , 0.10393212, 0.07607083, 0.13112448, 0.17474626, 0.20069916, 0.15598393, 0.10185637, 0.05784926, -0.05693372, -0.11891161, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]])
- s2n(quantile, points)float64nan nan nan nan ... nan nan nan nan
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
array([[ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1.00257482, 1.00276965, 1. , 1. , 1.00013855, 1.00207229, 1.00111875, 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]])
- corr(quantile, points)float64nan nan nan nan ... nan nan nan nan
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
array([[ nan, nan, nan, nan, 0.42873614, nan, nan, nan, nan, nan, nan, 0.41233324, 0.39350353, 0.35137978, 0.34100569, 0.33814258, 0.39019571, 0.42916593, 0.40919336, 0.40873375, 0.41006522, 0.40210314, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.47277882, nan, nan, nan, nan, nan, nan, 0.45835814, 0.43285955, 0.38151291, 0.36827288, 0.37602764, 0.40863275, 0.45070761, 0.44741026, 0.45561319, 0.45928193, 0.44042307, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.51020451, nan, nan, nan, nan, nan, nan, 0.47959407, 0.45953293, 0.40745234, 0.38833479, 0.40298447, 0.43577924, 0.47121164, 0.475997 , 0.47816371, 0.49603308, 0.46355137, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.56871458, nan, nan, nan, nan, nan, nan, 0.50001191, 0.49257397, 0.44253347, 0.41277589, 0.43670306, 0.46916191, 0.49656792, 0.50334054, 0.51923725, 0.52180771, 0.51023543, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.60282518, nan, nan, nan, nan, nan, nan, 0.53823595, 0.53873247, 0.47414713, 0.44882437, 0.49713443, 0.52893887, 0.5295324 , 0.5296688 , 0.57895664, 0.6112888 , 0.54288507, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]])
- cols(points)float6419.93 19.89 19.85 ... 18.53 18.5
array([19.92500178, 19.88835035, 19.85169892, 19.81238114, 19.77147862, 19.73851892, 19.7008962 , 19.66231484, 19.62359362, 19.58399811, 19.54547378, 19.5071079 , 19.46995093, 19.43594006, 19.39875245, 19.36100312, 19.3232538 , 19.28614625, 19.24853337, 19.2076012 , 19.17000792, 19.13331961, 19.09629324, 19.06012855, 19.02214227, 18.98317774, 18.94562997, 18.90897854, 18.87222201, 18.83447269, 18.79416836, 18.75293799, 18.71613052, 18.67932304, 18.64251557, 18.60570809, 18.56890062, 18.53209314, 18.49528567])
- rows(points)float6411.57 12.57 13.57 ... 48.55 49.54
array([11.571733 , 12.57106111, 13.57038922, 14.56961163, 15.56876812, 16.5682248 , 17.56751458, 18.56677004, 19.56602003, 20.56523583, 21.56449344, 22.5637572 , 23.56306479, 24.56248625, 25.56179366, 26.5610809 , 27.56036814, 28.55967933, 29.55897037, 30.5581323 , 31.55742304, 32.55674964, 33.55606381, 34.55540965, 35.55468792, 36.55392841, 37.55322251, 38.55255062, 39.55187482, 40.55116206, 41.55034749, 42.54949569, 43.54881807, 44.54814045, 45.54746283, 46.5467852 , 47.54610758, 48.54542996, 49.54475234])
- v_dir(points)float64-4.676 -4.676 ... -4.676 -4.676
- standard_name :
- river_flow_angle
- long_name :
- Angle of river flow in radians from North
- units :
- rad
array([-4.67572934, -4.67572934, -4.67439512, -4.67226783, -4.67544905, -4.67709031, -4.67427767, -4.67372806, -4.67322059, -4.67331912, -4.6739344 , -4.67461853, -4.67679747, -4.67678216, -4.67491172, -4.67463069, -4.6749518 , -4.67502004, -4.67310627, -4.67311606, -4.67523959, -4.67552328, -4.67578527, -4.67530498, -4.67390407, -4.67412347, -4.67528085, -4.67567676, -4.67512742, -4.67335217, -4.67161025, -4.67336003, -4.67557319, -4.67557319, -4.67557319, -4.67557319, -4.67557319, -4.67557319, -4.67557319])
- v_eff_nofill(quantile, points)float64nan nan nan nan ... nan nan nan nan
- standard_name :
- velocity
- long_name :
- velocity in perpendicular direction of cross section, measured by angle in radians, measured from up-direction
- units :
- m s-1
array([[ nan, nan, nan, nan, 0.08817558, nan, nan, nan, nan, nan, nan, 0.16908752, 0.21378354, 0.22828428, 0.23402424, 0.25268286, 0.26461829, 0.21896381, 0.16072446, 0.08492762, 0.18113962, 0.17583999, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.31874494, nan, nan, nan, nan, nan, nan, 0.20826553, 0.23859791, 0.27024558, 0.26854449, 0.3028189 , 0.32994231, 0.30228314, 0.26419603, 0.20577908, 0.24448365, 0.23604627, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.47875787, nan, nan, nan, nan, nan, nan, 0.22953366, 0.27389612, 0.3125006 , 0.30836882, 0.35548882, 0.41513323, 0.4086417 , 0.36217125, 0.30050657, 0.29065494, 0.26931073, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.56950871, nan, nan, nan, nan, nan, nan, 0.25956284, 0.30467886, 0.38222214, 0.40345521, 0.42007315, 0.46980839, 0.48222595, 0.41928195, 0.35767269, 0.35086026, 0.30484111, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.65185309, nan, nan, nan, nan, nan, nan, 0.28902697, 0.37296602, 0.4580432 , 0.48646452, 0.48525209, 0.51159036, 0.52681691, 0.49104038, 0.43909011, 0.40983011, 0.35432252, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]])
- quantilePandasIndex
PandasIndex(Index([0.05, 0.25, 0.5, 0.75, 0.95], dtype='float64', name='quantile'))
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
You can see that all coordinates and variables now only have a quantile
and points
dimension. The point
dimension represents all bathymetry points. By default, quantiles [0.05, 0.25, 0.5, 0.75, 0.95] are derived, but this can also be modified with the quantile
parameter. During the sampling, the bathymetry points are resampled to more or less match the resolution of the velocimetry results, in order to get a dense enough sampling. You can also impose a resampling distance using
the distance
parameter. If you would set this to 0.1, then a velocity will be sampled each 0.1 meters. Because our velocimetry grid has a 0.13 m resolution, the distance will by default be 0.13. The variables v_eff_nofill
and v_dir
are added, which is the effective velocity scalar, and its angle, perpendicular to the cross-section direction. v_eff_nofill
at this stage may contain gaps because of unknown velocities in moments and locations where the mask methods did not provide
satisfactory samples.
Using .get_q
, missing velocities will be filled with a logarithmic profile fit, and depth integrated velocities [m2/s] computed. We apply this on both cross sections and then check out the results.
[5]:
ds_points_q = ds_points.transect.get_q(fill_method="log_interp")
ds_points_q2 = ds_points2.transect.get_q(fill_method="log_interp")
ds_points_q
[5]:
<xarray.Dataset> Size: 17kB Dimensions: (points: 39, quantile: 5) Coordinates: (12/13) xp (points) float64 312B 492.0 515.7 ... 1.235e+03 1.252e+03 yp (points) float64 312B 287.1 294.1 301.1 ... 502.5 507.6 512.6 xs (points) float64 312B 6.427e+05 6.427e+05 ... 6.427e+05 ys (points) float64 312B 8.304e+06 8.304e+06 ... 8.304e+06 lon (points) float64 312B 28.33 28.33 28.33 ... 28.33 28.33 28.33 lat (points) float64 312B -15.33 -15.33 -15.33 ... -15.33 -15.33 ... ... y (points) float64 312B 6.166 6.036 5.906 ... 1.489 1.359 1.229 xcoords (points) float64 312B 6.427e+05 6.427e+05 ... 6.427e+05 ycoords (points) float64 312B 8.304e+06 8.304e+06 ... 8.304e+06 scoords (points) float64 312B 0.0 0.13 0.26 0.39 ... 4.68 4.81 4.94 zcoords (points) float64 312B 1.182e+03 1.182e+03 ... 1.183e+03 * quantile (quantile) float64 40B 0.05 0.25 0.5 0.75 0.95 Dimensions without coordinates: points Data variables: v_x (quantile, points) float64 2kB nan nan nan nan ... nan nan nan v_y (quantile, points) float64 2kB nan nan nan nan ... nan nan nan s2n (quantile, points) float64 2kB nan nan nan nan ... nan nan nan corr (quantile, points) float64 2kB nan nan nan nan ... nan nan nan cols (points) float64 312B 19.93 19.89 19.85 ... 18.57 18.53 18.5 rows (points) float64 312B 11.57 12.57 13.57 ... 47.55 48.55 49.54 v_dir (points) float64 312B -4.676 -4.676 -4.674 ... -4.676 -4.676 v_eff_nofill (quantile, points) float64 2kB 0.0 nan nan nan ... 0.0 0.0 0.0 v_eff (quantile, points) float64 2kB 0.0 0.02224 0.05672 ... 0.0 0.0 q_nofill (quantile, points) float64 2kB 0.0 nan nan nan ... 0.0 0.0 0.0 q (quantile, points) float64 2kB 0.0 0.001656 ... 0.0 0.0 Attributes: camera_shape: [1080, 1920] camera_config: {\n "height": 1080,\n "width": 1920,\n "crs": "P... h_a: 0.0
- points: 39
- quantile: 5
- xp(points)float64492.0 515.7 ... 1.235e+03 1.252e+03
- axis :
- X
- long_name :
- column in camera perspective
- units :
- -
array([ 491.96849675, 515.74554673, 539.28089429, 562.59440578, 585.68411844, 608.49303463, 631.10424013, 653.49803912, 675.6724587 , 697.63593419, 719.37812275, 740.9083282 , 762.22172734, 783.30634041, 804.21224281, 824.92321424, 845.43781861, 865.75368875, 885.882615 , 905.85033113, 925.60559226, 945.17026965, 964.55712362, 983.7584016 , 1002.79958346, 1021.67840564, 1040.37693127, 1058.89880116, 1077.2551038 , 1095.45649908, 1113.51966885, 1131.43219625, 1149.14597885, 1166.70405633, 1184.10847661, 1201.3612775 , 1218.46438105, 1235.4197276 , 1252.22926685])
- yp(points)float64287.1 294.1 301.1 ... 507.6 512.6
- axis :
- Y
- long_name :
- row in camera perspective
- units :
- -
array([287.06069404, 294.12937077, 301.12665793, 308.00211169, 314.77882468, 321.63961476, 328.34420774, 334.96503092, 341.51857452, 347.99238053, 354.42323554, 360.79505306, 367.12747981, 373.45527629, 379.66648101, 385.80903619, 391.89373753, 397.93248118, 403.90615475, 409.76799494, 415.63224803, 421.45758939, 427.22387032, 432.95168168, 438.59757363, 444.17284166, 449.7162139 , 455.22455046, 460.68210629, 466.07543954, 471.38065192, 476.62499604, 481.89287494, 487.11479597, 492.29139165, 497.42320476, 502.51084915, 507.55486587, 512.55581921])
- xs(points)float646.427e+05 6.427e+05 ... 6.427e+05
- axis :
- X
- long_name :
- x-coordinate in Cartesian system
- units :
- m
array([642732.215 , 642732.3428526 , 642732.47070519, 642732.59849397, 642732.72624444, 642732.85418309, 642732.98201246, 642733.10981936, 642733.23762293, 642733.36540564, 642733.4932139 , 642733.62102593, 642733.74886636, 642733.87678068, 642734.00462051, 642734.13244717, 642734.26027383, 642734.38811566, 642734.51594538, 642734.64369599, 642734.77152604, 642734.89937774, 642735.02722148, 642735.15508551, 642735.28290656, 642735.41070432, 642735.53853566, 642735.66638825, 642735.79423836, 642735.92206502, 642736.04983048, 642736.17757371, 642736.30542262, 642736.43327154, 642736.56112045, 642736.68896937, 642736.81681828, 642736.9446672 , 642737.07251611])
- ys(points)float648.304e+06 8.304e+06 ... 8.304e+06
- axis :
- Y
- long_name :
- y-coordinate in Cartesian system
- units :
- m
array([8304295.84700001, 8304295.82346886, 8304295.7999377 , 8304295.77606557, 8304295.75199084, 8304295.72893213, 8304295.70527676, 8304295.68149873, 8304295.65770282, 8304295.63379509, 8304295.61002435, 8304295.58627388, 8304295.56267809, 8304295.53948484, 8304295.51588511, 8304295.4922135 , 8304295.4685419 , 8304295.4449524 , 8304295.42129827, 8304295.39721962, 8304295.37356803, 8304295.35003216, 8304295.32645304, 8304295.30298416, 8304295.27928225, 8304295.25545522, 8304295.23180941, 8304295.20827826, 8304295.18473366, 8304295.16106206, 8304295.13706372, 8304295.11294697, 8304295.08939586, 8304295.06584474, 8304295.04229363, 8304295.01874251, 8304294.9951914 , 8304294.97164028, 8304294.94808916])
- lon(points)float6428.33 28.33 28.33 ... 28.33 28.33
- long_name :
- longitude
- units :
- degrees_east
array([28.32963233, 28.32963352, 28.32963471, 28.3296359 , 28.32963709, 28.32963829, 28.32963948, 28.32964067, 28.32964186, 28.32964305, 28.32964424, 28.32964544, 28.32964663, 28.32964782, 28.32964901, 28.3296502 , 28.3296514 , 28.32965259, 28.32965378, 28.32965497, 28.32965616, 28.32965736, 28.32965855, 28.32965974, 28.32966093, 28.32966212, 28.32966332, 28.32966451, 28.3296657 , 28.32966689, 28.32966808, 28.32966927, 28.32967047, 28.32967166, 28.32967285, 28.32967404, 28.32967523, 28.32967643, 28.32967762])
- lat(points)float64-15.33 -15.33 ... -15.33 -15.33
- long_name :
- latitude
- units :
- degrees_north
array([-15.33397933, -15.33397954, -15.33397974, -15.33397995, -15.33398016, -15.33398037, -15.33398057, -15.33398078, -15.33398099, -15.3339812 , -15.3339814 , -15.33398161, -15.33398182, -15.33398202, -15.33398223, -15.33398243, -15.33398264, -15.33398285, -15.33398305, -15.33398326, -15.33398347, -15.33398368, -15.33398388, -15.33398409, -15.33398429, -15.3339845 , -15.33398471, -15.33398492, -15.33398512, -15.33398533, -15.33398554, -15.33398575, -15.33398595, -15.33398616, -15.33398637, -15.33398657, -15.33398678, -15.33398698, -15.33398719])
- x(points)float642.655 2.65 2.646 ... 2.474 2.469
array([2.65525023, 2.65048555, 2.64572086, 2.64060955, 2.63529222, 2.63100746, 2.62611651, 2.62110093, 2.61606717, 2.61091975, 2.60591159, 2.60092403, 2.59609362, 2.59167221, 2.58683782, 2.58193041, 2.57702299, 2.57219901, 2.56730934, 2.56198816, 2.55710103, 2.55233155, 2.54751812, 2.54281671, 2.53787849, 2.53281311, 2.5279319 , 2.52316721, 2.51838886, 2.51348145, 2.50824189, 2.50288194, 2.49809697, 2.493312 , 2.48852702, 2.48374205, 2.47895708, 2.47417211, 2.46938714])
- y(points)float646.166 6.036 5.906 ... 1.359 1.229
array([6.16567471, 6.03576206, 5.9058494 , 5.77595049, 5.64606014, 5.51613078, 5.38622311, 5.25631989, 5.1264174 , 4.99651934, 4.86661585, 4.73671156, 4.60680158, 4.47687679, 4.34696682, 4.21705948, 4.08715214, 3.95724169, 3.82733385, 3.6974428 , 3.56753501, 3.43762255, 3.3077117 , 3.17779674, 3.04789057, 2.91798931, 2.78808107, 2.65816842, 2.52825627, 2.39834893, 2.26845483, 2.13856556, 2.00865365, 1.87874174, 1.74882983, 1.61891792, 1.48900601, 1.35909411, 1.2291822 ])
- xcoords(points)float646.427e+05 6.427e+05 ... 6.427e+05
array([642732.215 , 642732.34285259, 642732.47070518, 642732.59849397, 642732.72624444, 642732.85418308, 642732.98201245, 642733.10981936, 642733.23762293, 642733.36540563, 642733.49321389, 642733.62102592, 642733.74886635, 642733.87678067, 642734.0046205 , 642734.13244716, 642734.26027382, 642734.38811565, 642734.51594537, 642734.64369598, 642734.77152603, 642734.89937773, 642735.02722147, 642735.1550855 , 642735.28290654, 642735.41070431, 642735.53853565, 642735.66638824, 642735.79423835, 642735.92206501, 642736.04983046, 642736.17757369, 642736.30542261, 642736.43327152, 642736.56112044, 642736.68896935, 642736.81681827, 642736.94466718, 642737.0725161 ])
- ycoords(points)float648.304e+06 8.304e+06 ... 8.304e+06
array([8304295.847 , 8304295.82346885, 8304295.7999377 , 8304295.77606556, 8304295.75199083, 8304295.72893212, 8304295.70527675, 8304295.68149872, 8304295.65770281, 8304295.63379508, 8304295.61002434, 8304295.58627387, 8304295.56267808, 8304295.53948482, 8304295.51588509, 8304295.49221349, 8304295.46854188, 8304295.44495238, 8304295.42129826, 8304295.39721961, 8304295.37356801, 8304295.35003214, 8304295.32645302, 8304295.30298415, 8304295.27928223, 8304295.2554552 , 8304295.23180939, 8304295.20827824, 8304295.18473364, 8304295.16106204, 8304295.1370637 , 8304295.11294695, 8304295.08939584, 8304295.06584472, 8304295.0422936 , 8304295.01874249, 8304294.99519137, 8304294.97164026, 8304294.94808914])
- scoords(points)float640.0 0.13 0.26 ... 4.68 4.81 4.94
array([0. , 0.13, 0.26, 0.39, 0.52, 0.65, 0.78, 0.91, 1.04, 1.17, 1.3 , 1.43, 1.56, 1.69, 1.82, 1.95, 2.08, 2.21, 2.34, 2.47, 2.6 , 2.73, 2.86, 2.99, 3.12, 3.25, 3.38, 3.51, 3.64, 3.77, 3.9 , 4.03, 4.16, 4.29, 4.42, 4.55, 4.68, 4.81, 4.94])
- zcoords(points)float641.182e+03 1.182e+03 ... 1.183e+03
array([1182.3 , 1182.11724139, 1182.04766272, 1182.02136012, 1182.00426783, 1182.0288142 , 1182.05395595, 1182.07886069, 1182.1 , 1182.1 , 1182.1 , 1182.1 , 1182.1 , 1182.1 , 1182.07344832, 1182.04145034, 1182.00995529, 1181.97707483, 1181.9434698 , 1181.91002723, 1181.91869847, 1181.94585887, 1181.97331208, 1182. , 1182. , 1182. , 1182.07595305, 1182.19360881, 1182.30485649, 1182.35558135, 1182.40611729, 1182.45654225, 1182.50700892, 1182.5574756 , 1182.60794228, 1182.65840895, 1182.70887563, 1182.75934231, 1182.80980899])
- quantile(quantile)float640.05 0.25 0.5 0.75 0.95
array([0.05, 0.25, 0.5 , 0.75, 0.95])
- v_x(quantile, points)float64nan nan nan nan ... nan nan nan nan
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
array([[ nan, nan, nan, nan, 0.08639699, nan, nan, nan, nan, nan, nan, 0.17031846, 0.21440701, 0.22673029, 0.23322227, 0.25430978, 0.26647386, 0.22043808, 0.16107555, 0.07991675, 0.17314457, 0.16577959, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.31837207, nan, nan, nan, nan, nan, nan, 0.21024651, 0.24013688, 0.27037976, 0.26929555, 0.30550776, 0.33299914, 0.30500927, 0.26551842, 0.20316452, 0.23810597, 0.22829523, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.47957168, nan, nan, nan, nan, nan, nan, 0.23214022, 0.2759091 , 0.31364251, 0.31030323, 0.35949721, 0.41953946, 0.41225203, 0.36440482, 0.29994709, 0.28550313, 0.26278804, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.57224314, nan, nan, nan, nan, nan, nan, 0.26305541, 0.30719195, 0.38409759, 0.40652433, 0.4254107 , 0.47594587, 0.48711336, 0.42244001, 0.35852898, 0.34720895, 0.29926047, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.6585597 , nan, nan, nan, nan, nan, nan, 0.2943483 , 0.37690303, 0.4610435 , 0.49172284, 0.49219947, 0.5194662 , 0.53301662, 0.49542274, 0.44170202, 0.40799705, 0.35017769, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]])
- v_y(quantile, points)float64nan nan nan nan ... nan nan nan nan
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
array([[ nan, nan, nan, nan, -0.04975522, nan, nan, nan, nan, nan, nan, 0.02938081, 0.01370522, -0.04768946, -0.02577469, 0.0382964 , 0.04458772, 0.03534167, 0.00577576, -0.12919334, -0.21847965, -0.27601106, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, -0.01597724, nan, nan, nan, nan, nan, nan, 0.04848941, 0.03897506, -0.00104529, 0.01499823, 0.06546107, 0.0754373 , 0.06726933, 0.02845647, -0.07058136, -0.17613938, -0.214507 , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.01317713, nan, nan, nan, nan, nan, nan, 0.0646425 , 0.05165906, 0.02649235, 0.04581243, 0.09939644, 0.10987008, 0.08893217, 0.0497153 , -0.02014032, -0.14401374, -0.18181562, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.06347002, nan, nan, nan, nan, nan, nan, 0.08752184, 0.06515682, 0.04584315, 0.07429326, 0.13336247, 0.15506902, 0.12171582, 0.07211554, 0.01476788, -0.10475965, -0.1569285 , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.16943065, nan, nan, nan, nan, nan, nan, 0.1353601 , 0.10393212, 0.07607083, 0.13112448, 0.17474626, 0.20069916, 0.15598393, 0.10185637, 0.05784926, -0.05693372, -0.11891161, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]])
- s2n(quantile, points)float64nan nan nan nan ... nan nan nan nan
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
array([[ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 1. , nan, nan, nan, nan, nan, nan, 1.00257482, 1.00276965, 1. , 1. , 1.00013855, 1.00207229, 1.00111875, 1. , 1. , 1. , 1. , nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]])
- corr(quantile, points)float64nan nan nan nan ... nan nan nan nan
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
array([[ nan, nan, nan, nan, 0.42873614, nan, nan, nan, nan, nan, nan, 0.41233324, 0.39350353, 0.35137978, 0.34100569, 0.33814258, 0.39019571, 0.42916593, 0.40919336, 0.40873375, 0.41006522, 0.40210314, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.47277882, nan, nan, nan, nan, nan, nan, 0.45835814, 0.43285955, 0.38151291, 0.36827288, 0.37602764, 0.40863275, 0.45070761, 0.44741026, 0.45561319, 0.45928193, 0.44042307, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.51020451, nan, nan, nan, nan, nan, nan, 0.47959407, 0.45953293, 0.40745234, 0.38833479, 0.40298447, 0.43577924, 0.47121164, 0.475997 , 0.47816371, 0.49603308, 0.46355137, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.56871458, nan, nan, nan, nan, nan, nan, 0.50001191, 0.49257397, 0.44253347, 0.41277589, 0.43670306, 0.46916191, 0.49656792, 0.50334054, 0.51923725, 0.52180771, 0.51023543, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan], [ nan, nan, nan, nan, 0.60282518, nan, nan, nan, nan, nan, nan, 0.53823595, 0.53873247, 0.47414713, 0.44882437, 0.49713443, 0.52893887, 0.5295324 , 0.5296688 , 0.57895664, 0.6112888 , 0.54288507, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]])
- cols(points)float6419.93 19.89 19.85 ... 18.53 18.5
array([19.92500178, 19.88835035, 19.85169892, 19.81238114, 19.77147862, 19.73851892, 19.7008962 , 19.66231484, 19.62359362, 19.58399811, 19.54547378, 19.5071079 , 19.46995093, 19.43594006, 19.39875245, 19.36100312, 19.3232538 , 19.28614625, 19.24853337, 19.2076012 , 19.17000792, 19.13331961, 19.09629324, 19.06012855, 19.02214227, 18.98317774, 18.94562997, 18.90897854, 18.87222201, 18.83447269, 18.79416836, 18.75293799, 18.71613052, 18.67932304, 18.64251557, 18.60570809, 18.56890062, 18.53209314, 18.49528567])
- rows(points)float6411.57 12.57 13.57 ... 48.55 49.54
array([11.571733 , 12.57106111, 13.57038922, 14.56961163, 15.56876812, 16.5682248 , 17.56751458, 18.56677004, 19.56602003, 20.56523583, 21.56449344, 22.5637572 , 23.56306479, 24.56248625, 25.56179366, 26.5610809 , 27.56036814, 28.55967933, 29.55897037, 30.5581323 , 31.55742304, 32.55674964, 33.55606381, 34.55540965, 35.55468792, 36.55392841, 37.55322251, 38.55255062, 39.55187482, 40.55116206, 41.55034749, 42.54949569, 43.54881807, 44.54814045, 45.54746283, 46.5467852 , 47.54610758, 48.54542996, 49.54475234])
- v_dir(points)float64-4.676 -4.676 ... -4.676 -4.676
- standard_name :
- river_flow_angle
- long_name :
- Angle of river flow in radians from North
- units :
- rad
array([-4.67572934, -4.67572934, -4.67439512, -4.67226783, -4.67544905, -4.67709031, -4.67427767, -4.67372806, -4.67322059, -4.67331912, -4.6739344 , -4.67461853, -4.67679747, -4.67678216, -4.67491172, -4.67463069, -4.6749518 , -4.67502004, -4.67310627, -4.67311606, -4.67523959, -4.67552328, -4.67578527, -4.67530498, -4.67390407, -4.67412347, -4.67528085, -4.67567676, -4.67512742, -4.67335217, -4.67161025, -4.67336003, -4.67557319, -4.67557319, -4.67557319, -4.67557319, -4.67557319, -4.67557319, -4.67557319])
- v_eff_nofill(quantile, points)float640.0 nan nan nan ... 0.0 0.0 0.0 0.0
- standard_name :
- velocity
- long_name :
- velocity in perpendicular direction of cross section, measured by angle in radians, measured from up-direction
- units :
- m s-1
array([[0. , nan, nan, nan, 0.08817558, nan, nan, nan, nan, nan, nan, 0.16908752, 0.21378354, 0.22828428, 0.23402424, 0.25268286, 0.26461829, 0.21896381, 0.16072446, 0.08492762, 0.18113962, 0.17583999, nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , nan, nan, nan, 0.31874494, nan, nan, nan, nan, nan, nan, 0.20826553, 0.23859791, 0.27024558, 0.26854449, 0.3028189 , 0.32994231, 0.30228314, 0.26419603, 0.20577908, 0.24448365, 0.23604627, nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , nan, nan, nan, 0.47875787, nan, nan, nan, nan, nan, nan, 0.22953366, 0.27389612, 0.3125006 , 0.30836882, 0.35548882, 0.41513323, 0.4086417 , 0.36217125, 0.30050657, 0.29065494, 0.26931073, nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , nan, nan, nan, 0.56950871, nan, nan, nan, nan, nan, nan, 0.25956284, 0.30467886, 0.38222214, 0.40345521, 0.42007315, 0.46980839, 0.48222595, 0.41928195, 0.35767269, 0.35086026, 0.30484111, nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , nan, nan, nan, 0.65185309, nan, nan, nan, nan, nan, nan, 0.28902697, 0.37296602, 0.4580432 , 0.48646452, 0.48525209, 0.51159036, 0.52681691, 0.49104038, 0.43909011, 0.40983011, 0.35432252, nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ]])
- v_eff(quantile, points)float640.0 0.02224 0.05672 ... 0.0 0.0 0.0
- standard_name :
- velocity
- long_name :
- velocity in perpendicular direction of cross section, measured by angle in radians, measured from up-direction
- units :
- m s-1
array([[0. , 0.02223675, 0.05671689, 0.07487398, 0.08817558, 0.10125593, 0.11365495, 0.12547749, 0.13684926, 0.14791528, 0.15863413, 0.16908752, 0.21378354, 0.22828428, 0.23402424, 0.25268286, 0.26461829, 0.21896381, 0.16072446, 0.08492762, 0.18113962, 0.17583999, 0.16400474, 0.15009652, 0.13448481, 0.11569975, 0.08284174, 0.02063667, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , 0.08038338, 0.20502526, 0.27066111, 0.31874494, 0.32483631, 0.32128613, 0.30979103, 0.29178058, 0.26842625, 0.24034167, 0.20826553, 0.23859791, 0.27024558, 0.26854449, 0.3028189 , 0.32994231, 0.30228314, 0.26419603, 0.20577908, 0.24448365, 0.23604627, 0.22015872, 0.20148844, 0.1805314 , 0.15531447, 0.11120613, 0.02770252, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , 0.12073659, 0.30794985, 0.40653551, 0.47875787, 0.47939369, 0.46406418, 0.43558999, 0.3963199 , 0.34814724, 0.29215144, 0.22953366, 0.27389612, 0.3125006 , 0.30836882, 0.35548882, 0.41513323, 0.4086417 , 0.36217125, 0.30050657, 0.29065494, 0.26931073, 0.25118425, 0.22988288, 0.20597251, 0.17720192, 0.12687768, 0.03160645, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , 0.14362279, 0.36632322, 0.48359626, 0.56950871, 0.56888708, 0.54903368, 0.5133479 , 0.46465635, 0.40523294, 0.3363872 , 0.25956284, 0.30467886, 0.38222214, 0.40345521, 0.42007315, 0.46980839, 0.48222595, 0.41928195, 0.35767269, 0.35086026, 0.30484111, 0.28432319, 0.26021151, 0.23314662, 0.20058031, 0.14361675, 0.03577631, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , 0.16438898, 0.41928933, 0.55351869, 0.65185309, 0.65031709, 0.62662493, 0.5846939 , 0.52777883, 0.45849535, 0.37835763, 0.28902697, 0.37296602, 0.4580432 , 0.48646452, 0.48525209, 0.51159036, 0.52681691, 0.49104038, 0.43909011, 0.40983011, 0.35432252, 0.33047416, 0.30244871, 0.27099068, 0.23313824, 0.16692844, 0.04158348, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ]])
- q_nofill(quantile, points)float640.0 nan nan nan ... 0.0 0.0 0.0 0.0
- standard_name :
- velocity_depth
- long_name :
- velocity averaged over depth
- units :
- m2 s-1
array([[0. , nan, nan, nan, 0.01553292, nan, nan, nan, nan, nan, nan, 0.01521788, 0.01924052, 0.02054559, 0.02665454, 0.0360565 , 0.04526038, 0.04393129, 0.03710761, 0.02216403, 0.04585937, 0.04021936, nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , nan, nan, nan, 0.05614977, nan, nan, nan, nan, nan, nan, 0.0187439 , 0.02147381, 0.0243221 , 0.03058628, 0.04321065, 0.05643341, 0.06064787, 0.06099683, 0.0537033 , 0.06189626, 0.05399016, nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , nan, nan, nan, 0.08433749, nan, nan, nan, nan, nan, nan, 0.02065803, 0.02465065, 0.02812505, 0.03512213, 0.05072637, 0.07100449, 0.08198687, 0.08361708, 0.07842485, 0.07358551, 0.06159864, nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , nan, nan, nan, 0.10032406, nan, nan, nan, nan, nan, nan, 0.02336066, 0.0274211 , 0.03439999, 0.04595214, 0.05994221, 0.08035614, 0.09675027, 0.09680263, 0.09334381, 0.08882777, 0.0697254 , nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , nan, nan, nan, 0.11482976, nan, nan, nan, nan, nan, nan, 0.02601243, 0.03356694, 0.04122389, 0.05540661, 0.0692429 , 0.08750254, 0.10569667, 0.11337002, 0.11459176, 0.10375725, 0.08104313, nan, nan, nan, nan, nan, nan, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ]])
- q(quantile, points)float640.0 0.001656 0.007776 ... 0.0 0.0
- standard_name :
- velocity_depth
- long_name :
- velocity averaged over depth
- units :
- m2 s-1
array([[0. , 0.00165625, 0.00777609, 0.01203793, 0.01553292, 0.01560022, 0.01493877, 0.01368023, 0.01231643, 0.01331238, 0.01427707, 0.01521788, 0.01924052, 0.02054559, 0.02665454, 0.0360565 , 0.04526038, 0.04393129, 0.03710761, 0.02216403, 0.04585937, 0.04021936, 0.0334601 , 0.02701737, 0.02420727, 0.02082595, 0.00924864, 0.0001187 , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , 0.00598718, 0.02810969, 0.04351578, 0.05614977, 0.05004663, 0.04222974, 0.03377508, 0.02626025, 0.02415836, 0.02163075, 0.0187439 , 0.02147381, 0.0243221 , 0.03058628, 0.04321065, 0.05643341, 0.06064787, 0.06099683, 0.0537033 , 0.06189626, 0.05399016, 0.04491659, 0.03626792, 0.03249565, 0.0279566 , 0.0124153 , 0.00015935, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , 0.00899279, 0.04222102, 0.06536111, 0.08433749, 0.07385885, 0.06099643, 0.04749036, 0.03566879, 0.03133325, 0.02629363, 0.02065803, 0.02465065, 0.02812505, 0.03512213, 0.05072637, 0.07100449, 0.08198687, 0.08361708, 0.07842485, 0.07358551, 0.06159864, 0.05124639, 0.04137892, 0.03707505, 0.03189635, 0.01416491, 0.0001818 , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , 0.01069742, 0.05022421, 0.07775062, 0.10032406, 0.08764685, 0.07216479, 0.05596795, 0.04181907, 0.03647096, 0.03027485, 0.02336066, 0.0274211 , 0.03439999, 0.04595214, 0.05994221, 0.08035614, 0.09675027, 0.09680263, 0.09334381, 0.08882777, 0.0697254 , 0.05800737, 0.04683807, 0.04196639, 0.03610446, 0.0160337 , 0.00020579, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [0. , 0.01224414, 0.05748606, 0.08899246, 0.11482976, 0.10019255, 0.08236336, 0.06374647, 0.04750009, 0.04126458, 0.03405219, 0.02601243, 0.03356694, 0.04122389, 0.05540661, 0.0692429 , 0.08750254, 0.10569667, 0.11337002, 0.11459176, 0.10375725, 0.08104313, 0.06742305, 0.05444077, 0.04877832, 0.04196488, 0.01863627, 0.00023919, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ]])
- quantilePandasIndex
PandasIndex(Index([0.05, 0.25, 0.5, 0.75, 0.95], dtype='float64', name='quantile'))
- camera_shape :
- [1080, 1920]
- camera_config :
- { "height": 1080, "width": 1920, "crs": "PROJCRS[\"WGS 84 / UTM zone 35S\",BASEGEOGCRS[\"WGS 84\",ENSEMBLE[\"World Geodetic System 1984 ensemble\",MEMBER[\"World Geodetic System 1984 (Transit)\"],MEMBER[\"World Geodetic System 1984 (G730)\"],MEMBER[\"World Geodetic System 1984 (G873)\"],MEMBER[\"World Geodetic System 1984 (G1150)\"],MEMBER[\"World Geodetic System 1984 (G1674)\"],MEMBER[\"World Geodetic System 1984 (G1762)\"],MEMBER[\"World Geodetic System 1984 (G2139)\"],ELLIPSOID[\"WGS 84\",6378137,298.257223563,LENGTHUNIT[\"metre\",1]],ENSEMBLEACCURACY[2.0]],PRIMEM[\"Greenwich\",0,ANGLEUNIT[\"degree\",0.0174532925199433]],ID[\"EPSG\",4326]],CONVERSION[\"UTM zone 35S\",METHOD[\"Transverse Mercator\",ID[\"EPSG\",9807]],PARAMETER[\"Latitude of natural origin\",0,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8801]],PARAMETER[\"Longitude of natural origin\",27,ANGLEUNIT[\"degree\",0.0174532925199433],ID[\"EPSG\",8802]],PARAMETER[\"Scale factor at natural origin\",0.9996,SCALEUNIT[\"unity\",1],ID[\"EPSG\",8805]],PARAMETER[\"False easting\",500000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8806]],PARAMETER[\"False northing\",10000000,LENGTHUNIT[\"metre\",1],ID[\"EPSG\",8807]]],CS[Cartesian,2],AXIS[\"(E)\",east,ORDER[1],LENGTHUNIT[\"metre\",1]],AXIS[\"(N)\",north,ORDER[2],LENGTHUNIT[\"metre\",1]],USAGE[SCOPE[\"Engineering survey, topographic mapping.\"],AREA[\"Between 24\u00b0E and 30\u00b0E, southern hemisphere between 80\u00b0S and equator, onshore and offshore. Botswana. Burundi. Democratic Republic of the Congo (Zaire). Rwanda. South Africa. Tanzania. Uganda. Zambia. Zimbabwe.\"],BBOX[-80,24,0,30]],ID[\"EPSG\",32735]]", "resolution": 0.01, "lens_position": null, "gcps": { "src": [ [ 1421.0, 1001.0 ], [ 1251.0, 460.0 ], [ 421.0, 432.0 ], [ 470.0, 607.0 ] ], "dst": [ [ 642735.8076, 8304292.119 ], [ 642737.5823, 8304295.593 ], [ 642732.7864, 8304298.425 ], [ 642732.6705, 8304296.858 ] ], "h_ref": 0.0, "z_0": 1182.2 }, "window_size": 25, "is_nadir": false, "camera_matrix": [ [ 1552.16064453125, 0.0, 960.0 ], [ 0.0, 1552.16064453125, 540.0 ], [ 0.0, 0.0, 1.0 ] ], "dist_coeffs": [ [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ], [ 0.0 ] ], "bbox": "POLYGON ((642730.2341900971 8304293.357399672, 642731.5015509361 8304302.01513012, 642739.268771966 8304300.878126396, 642738.0014111269 8304292.220395949, 642730.2341900971 8304293.357399672))" }
- h_a :
- 0.0
Now we have variables v_eff
with velocities (filled with zeros where no data was found, but log profiles are also possible), and q
and q_nofill
which hold the depth integrated velocities. During depth integration the default assumption is that the depth average velocities is 0.9 times the surface velocity. This can be controlled by the v_corr
parameter. Below, we make a quick plot of the q
results for both profiles.
[6]:
ax = plt.axes()
ds_points_q["v_eff"].isel(quantile=2).plot(ax=ax)
ds_points_q2["v_eff"].isel(quantile=2).plot(ax=ax)
plt.grid()
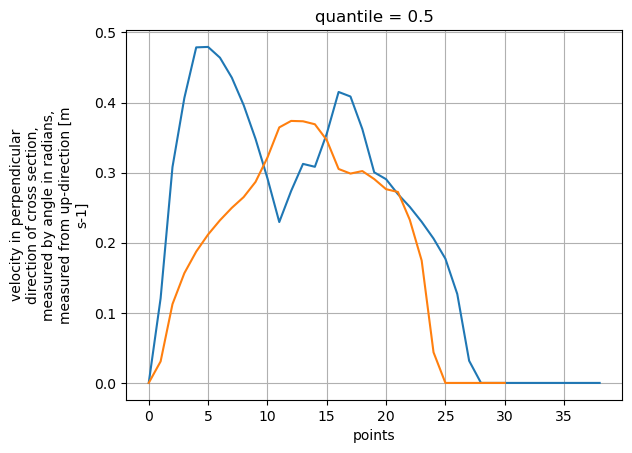
We can also plot the sampled surface velocities in combination with the velocity grid with bespoke plotting functions, giving intuitive graphics. We do this in the camera perspective below, similar to notebook 03.
[7]:
# plot the rgb frame first. We use the "camera" mode to plot the camera perspective.
norm = Normalize(vmin=0., vmax=0.6, clip=False)
p = da_rgb[0].frames.plot(mode="camera")
# extract mean velocity and plot in camera projection
ds.mean(dim="time", keep_attrs=True).velocimetry.plot(
ax=p.axes,
mode="camera",
cmap="rainbow",
scale=200,
width=0.001,
alpha=0.3,
norm=norm,
)
# plot velocimetry point results in camera projection
ds_points_q.isel(quantile=2).transect.plot(
ax=p.axes,
mode="camera",
cmap="rainbow",
scale=100,
width=0.003,
norm=norm,
)
ds_points_q2.isel(quantile=2).transect.plot(
ax=p.axes,
mode="camera",
cmap="rainbow",
scale=100,
width=0.003,
norm=norm,
add_colorbar=True
)
# store figure in a JPEG
p.axes.figure.savefig("ngwerere.jpg", dpi=200)
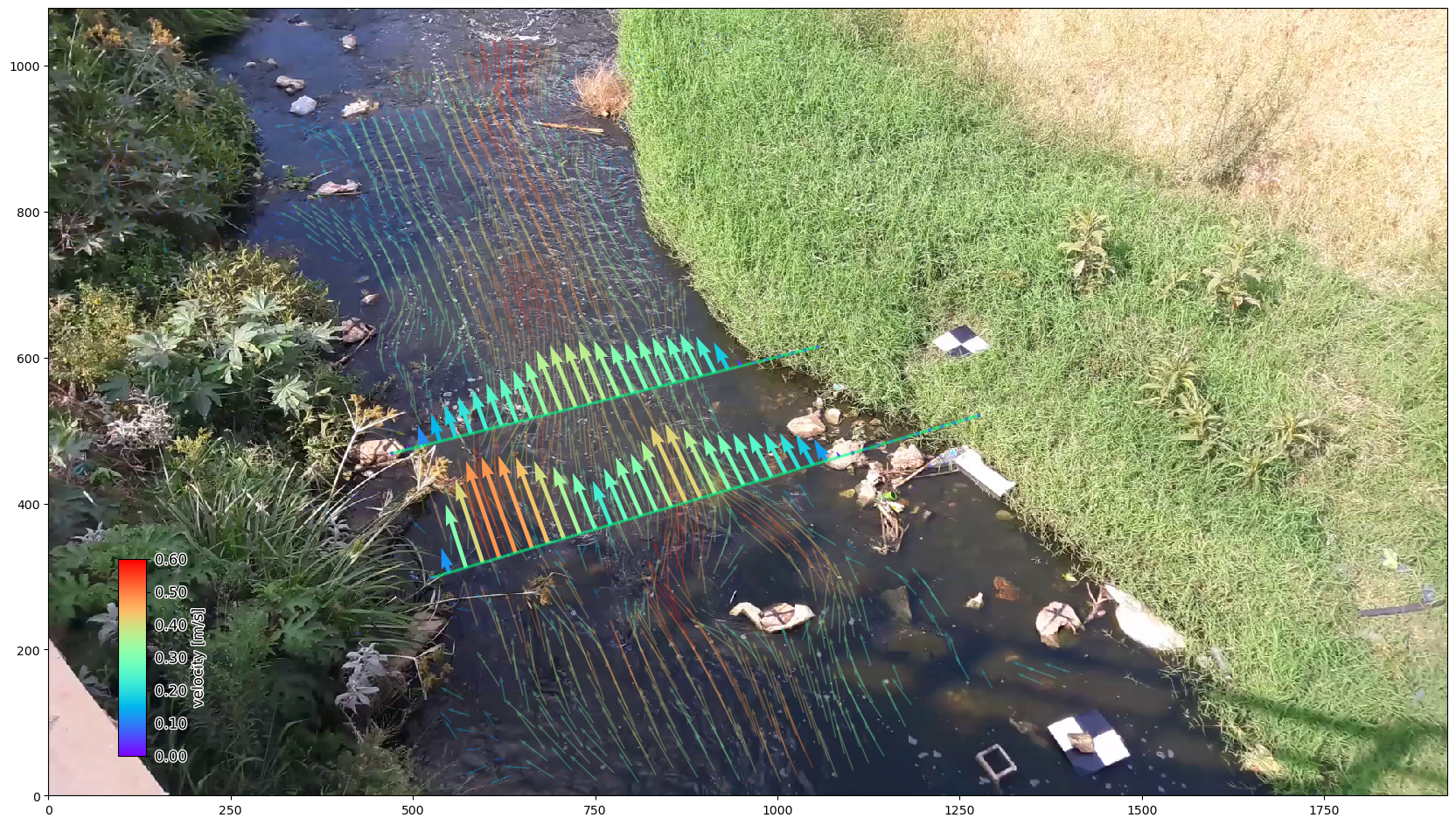
Of course we can also plot our results in a local projection and add a selected plotting style to it. Below this is shown with a streamplot as example.
[8]:
# again plot the projected background
from matplotlib.colors import Normalize
norm = Normalize(vmin=0, vmax=0.6, clip=False)
ds_mean = ds.mean(dim="time", keep_attrs=True)
p = da_rgb.frames.project()[0].frames.plot(mode="local")
# plot velocimetry point results in local projection
ds_points_q.isel(quantile=2).transect.plot(
ax=p.axes,
mode="local",
cmap="rainbow",
scale=10,
width=0.003,
norm=norm,
add_colorbar=True,
)
ds_points_q2.isel(quantile=2).transect.plot(
ax=p.axes,
mode="local",
cmap="rainbow",
scale=10,
width=0.003,
norm=norm,
add_colorbar=True,
)
# to ensure streamplot understands the directions correctly, all values must
# be flipped upside down and up-down velocities become down-up velocities.
ds_mean.velocimetry.plot.streamplot(
ax=p.axes,
mode="local",
density=3.,
minlength=0.05,
linewidth_scale=2,
cmap="rainbow",
norm=norm,
add_colorbar=True
)
/usr/share/miniconda/envs/pyorc-dev/lib/python3.10/site-packages/matplotlib/colors.py:2243: UserWarning: Warning: converting a masked element to nan.
dtype = np.min_scalar_type(value)
/usr/share/miniconda/envs/pyorc-dev/lib/python3.10/site-packages/matplotlib/colors.py:2250: UserWarning: Warning: converting a masked element to nan.
data = np.asarray(value)
[8]:
<matplotlib.collections.LineCollection at 0x7fd08494c850>
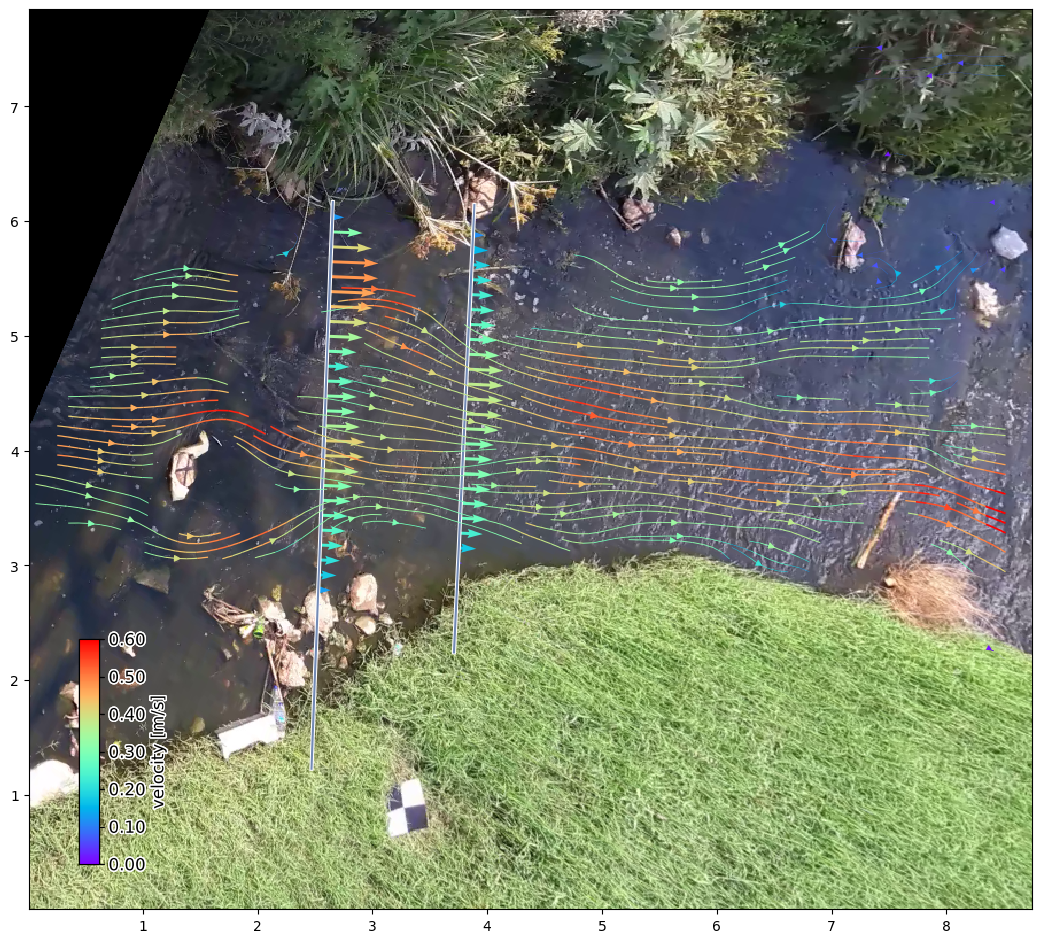
Finally, we can extract discharge estimates from the cross section
[9]:
ds_points_q.transect.get_river_flow()
print(ds_points_q["river_flow"])
ds_points_q2.transect.get_river_flow()
print(ds_points_q2["river_flow"])
<xarray.DataArray 'river_flow' (quantile: 5)> Size: 40B
array([0.07647424, 0.1263703 , 0.16405959, 0.19231923, 0.22172388])
Coordinates:
* quantile (quantile) float64 40B 0.05 0.25 0.5 0.75 0.95
Attributes:
standard_name: river_discharge
long_name: River Flow
units: m3 s-1
<xarray.DataArray 'river_flow' (quantile: 5)> Size: 40B
array([0.11772497, 0.14319452, 0.16357151, 0.18420046, 0.22154355])
Coordinates:
* quantile (quantile) float64 40B 0.05 0.25 0.5 0.75 0.95
Attributes:
standard_name: river_discharge
long_name: River Flow
units: m3 s-1
You can see that the different quantiles give very diverse values, but that even with this very shallow and difficult example, the flow estimates are quite close to each other. The bathymetry was recorded at only 10 cm accuracy and the site is far from uniform, and so not ideal for cross-sectional discharge observations. This can easily explain the differences between two sampled cross sections.
The diversity in quantile values can be because of many reasons and should be considered highly conservative. The real uncertainty is likely to be smaller in case velocities are well sampled. In case many velocities are filled in with interpolation, the uncertainty may also be larger. Reasons for variability are:
the integration assumes that all velocity estimates for the same quantile, occur entirely correlated. This is naturally not the case in reality. Instead, water may vary slightly in its path from moment to moment and therefore the quantiles are a very conservative estimate. Especially during very low and shallow flows with lots of variability in direction and scalar values (such as in this example) this may lead to quite wide variability.
Frames-per-second of a video are not as constant as we would like them to be and many cameras (in particular cheap IP cameras) are not good at keeping track of the time evolution per frame. This can cause a lot of variability which has nothing to do with real uncertainty.
pyorc
does read frame-specific timings in case frame rates are not constant.last but not least, remaining uncertainties in the PIV solution can cause variability.